Artificial Intelligence
- nekiristailgame
- Mar 8, 2020
- 4 min read
Dylan Ascencio
Nekiri’s Tail’s main mechanic involves avoiding the body-usurping Damned, which move around the level and attempt to chase the player when they spot them. That means that the Damned must have some sort of artificial intelligence - being able to “see” the player, being able to know where they can and cannot move, when to do certain other actions, and so on. Before this project, I had very little experience with Unreal Engine 4’s AI subsystem. Learning it was a challenge, but ultimately I came up with a system that was both effective and easy to modify.
Behavior Trees are one of the subsystems that Unreal Engine 4 provides for artificial intelligence. Trees are paired with a Blackboard, which contains variables that the Tree can read and modify. The Tree is made up of Behavior Tasks, which run whatever processes that Task is designed to do. As shown in the image above, the tree begins at the ROOT node and moves down to the first Selector node. From there, the Behavior Tree will move from left to right, attempting to execute each Task it encounters. The Tasks have three options here: return to the Behavior Tree with a success, return to the Tree with a failure, or don’t return to the Tree at all. They can evaluate any combination of conditions, whether from the Blackboard or the character’s pawn and controller objects, in order to decide which option to take.
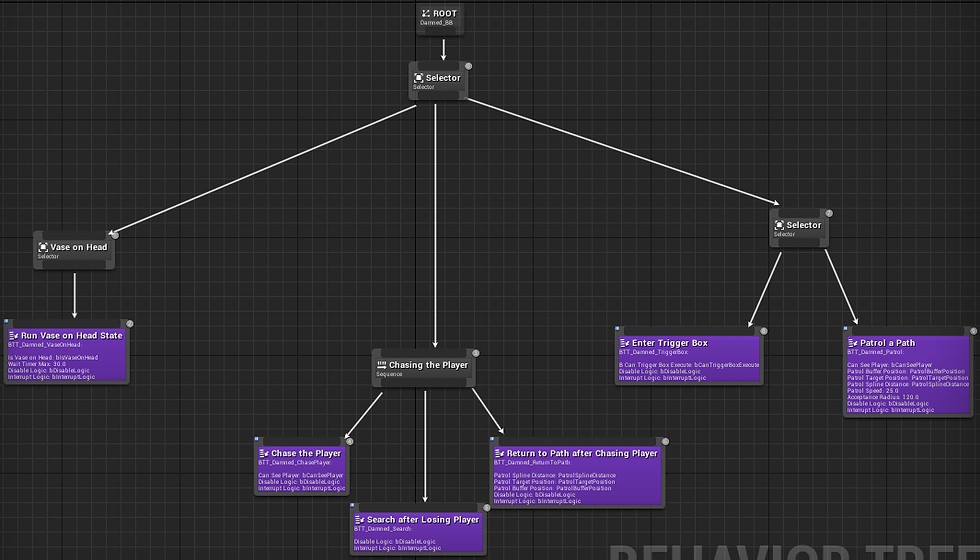
Returning a success or failure to the Behavior Tree causes it to do different things depending on the node above the Task. If the Task’s parent node is a Selector, then a failure causes the tree to continue checking Tasks from left to right, while a success forces the Tree to restart from the ROOT node. However, if the Task’s parent node is a Sequence, these conditions are reversed - a success allows the Tree to continue while a failure resets it.
What happens if a Task doesn’t return to the Behavior Tree? It begins to run its Tick() function - a function that runs once every frame. The Task will continue to tick until it finally returns something to the Behavior Tree. A ticking Behavior Task has the potential to become a complex state machine, managing the execution of parts of an action - for example, having states for beginning, looping, and ending a behavior. This is how my implementation of the Damned’s AI works.
All of the Tasks in the Damned’s Behavior Tree are derived from a common base Task. The base Task has prototypes for the following functions:
Decide if Performing Behavior: Called when the Behavior Tree attempts to execute the Task. The base implementation only checks the Blackboard’s DisableLogic boolean, which is enabled when AI should be disabled. Derivatives typically also add checks to various other variables, such as whether the Damned can see the player or whether it is colliding with a certain object.
CheckForInterrupt: Running before the Execute function described below, this function checks the Blackboard’s Interrupt Logic boolean and short circuits the Behavior Task’s execution if it is set. This allows the Task to be cancelled prematurely, before it finishes execution naturally. As an example, it can be used if an action with a higher priority must be executed while a lower priority Task is processing.
Execute: Execute() is attached to the base Task’s Tick() function and runs every frame. If a deriving Task passes its DecideIfPerformingBehavior() check, this function begins to run. Anything the Task needs to do to accomplish its behavior is defined here.
SetupTask: This is run when the Task has decided that it will begin to tick. It initializes local variables and ensures that the state of the Task is always the same when the action begins.
CleanupTask: The opposite of SetupTask() above. CleanupTask() is run right before the Task returns a success to the Behavior Tree, reverting any changes the Task might have made to the state of the Blackboard or character objects themselves.
A new behavior can be defined by deriving from the base Task object, implementing these functions with the desired functionality, and attaching the Task to the Behavior Tree. This reduces development time by a large factor. In my experience, new behaviors can be prototyped and implemented with this system in as little as two hours.
Example: Patrolling
I will demonstrate my AI system by showing how the Patrolling behavior implements all of the base Task’s functions. When the Damned patrol, they follow a spline path defined within the level.
DecideIfPerformingBehavior() checks if the Damned’s pawn has a valid path object assigned to its Path variable, comparing its value with None. It logically ANDs the result of that comparison with the base Task’s implementation of the function, which just returns the state of the DisableLogic boolean. Therefore, the result of this function boils down to “DisableLogic is not set AND Path variable is not None.”
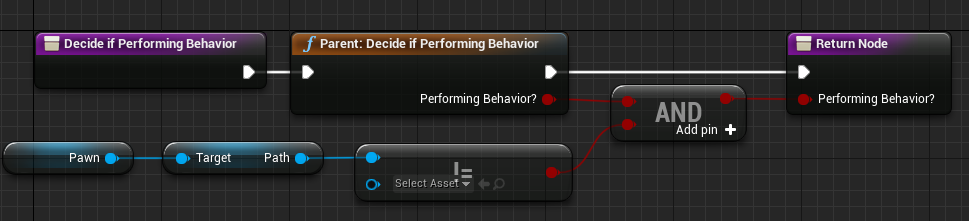
The patrolling behavior’s CheckForInterrupt() checks both the Blackboard’s InterruptLogic variable (from the base Task’s implementation) and whether the Damned can currently see the player. If either of those conditions are true, the Task will prematurely end, allowing the Behavior Tree to begin the Chase Player behavior.
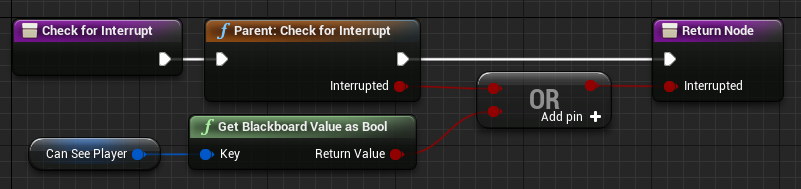
Before the Task begins to execute, it will use SetupTask() to set the Damned’s walking speed and get the first point along the path that it should move to.

Finally, the Execute() monitors the Damned’s location and checks if it is within a certain distance of its target point on the path. Once it is within that distance, it will set the Damned’s next target point via GetNextPoint() and return a success to the Behavior Tree by returning true to Tick(). Otherwise, it continues to loop by returning false.
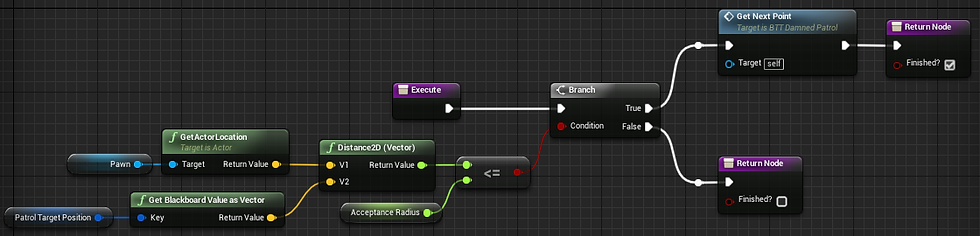
Unreal Engine 4’s AI subsystem is particularly flexible, allowing the user to configure its behavior however they like. I took advantage of this to create a system for Nekiri’s Tail that allows us to easily define new behaviors for our enemy characters. I don’t think that there is anything I would change about it right now - it fits our needs perfectly.
Comments